In the following article we extend the Tiny RTC module with a DS18B20 temperature sensor, which either already exists on the board or we simply add by soldering. This article is an extension of: Time and calendar functions with a real time clock module based on the DS1307 and an Arduino.
We wiring our Arduino as follows with the real-time module:
Zoom level:
Move over elements (parts, jumper cable etc.) for more information (or tap in touch mode)...
Arduino (Nano, Uno, Pro Mini) |
Arduino (Mega, Mega 2560) |
Tiny RTC-Modul DS1307 |
5V (VCC) | 5V (VCC) | VCC |
GND | GND | GND |
A4 (SDA) | D20 (SDA) | SDA |
A5 (SCL) | D21 (SCL) | SCL |
D2 | D2 | DS |
Sourcecode (Sketch):
In addition, we install three additional libraries (if not already present, of course):
- the RTClib by Jean-Claude Wippler
- the Arduino-Temperature-Control-Library by Miles Burton
- the OneWire-Bibliothek by Paul Stoffregen.
and then upload the following code to the Arduino:
// Tiny RTC (DS1307) #include <Wire.h> // I2C-Bibliothek einbinden #include "RTClib.h" // RTC-Bibliothek einbinden RTC_DS1307 RTC; // RTC Modul #include <OneWire.h> // OneWire-Bibliothek einbinden #include <DallasTemperature.h> // DS18B20-Bibliothek einbinden #define DS18B20_PIN 2 // Pin für DS18B20 definieren Arduino D2 OneWire oneWire(DS18B20_PIN); // OneWire Referenz setzen DallasTemperature sensors(&oneWire); // DS18B20 initialisieren void setup(void) { // Initialisiere I2C Wire.begin(); // Initialisiere RTC RTC.begin(); // Serielle Ausgabe starten Serial.begin(9600); // Begrüßungstext auf seriellem Monitor ausgeben Serial.println("Starte Datum, Zeit und Temperatur - blog.simtronyx.de"); // Prüfen ob RTC läuft if (! RTC.isrunning()) { // Aktuelles Datum und Zeit setzen, falls die Uhr noch nicht läuft RTC.adjust(DateTime(__DATE__, __TIME__)); Serial.println("Echtzeituhr wurde gestartet und auf Systemzeit gesetzt."); } else Serial.println("Echtzeituhr laeuft bereits."); sensors.begin(); // DS18B20 starten } void loop(){ DateTime now=RTC.now(); // aktuelle Zeit abrufen show_time_and_date(now); // Datum und Uhrzeit ausgeben sensors.requestTemperatures(); // Temperatursensor auslesen show_temperature(sensors.getTempCByIndex(0)); // Temperatur ausgeben delay(30000); // 30 Sekunden warten bis zur nächsten Ausgabe } // Wochentag ermitteln String get_day_of_week(uint8_t dow){ String dows=" "; switch(dow){ case 0: dows="So"; break; case 1: dows="Mo"; break; case 2: dows="Di"; break; case 3: dows="Mi"; break; case 4: dows="Do"; break; case 5: dows="Fr"; break; case 6: dows="Sa"; break; } return dows; } // Datum und Uhrzeit ausgeben void show_time_and_date(DateTime datetime){ // Wochentag, Tag.Monat.Jahr Serial.print(get_day_of_week(datetime.dayOfWeek())); Serial.print(", "); if(datetime.day()<10)Serial.print(0); Serial.print(datetime.day(),DEC); Serial.print("."); if(datetime.month()<10)Serial.print(0); Serial.print(datetime.month(),DEC); Serial.print("."); Serial.println(datetime.year(),DEC); // Stunde:Minute:Sekunde if(datetime.hour()<10)Serial.print(0); Serial.print(datetime.hour(),DEC); Serial.print(":"); if(datetime.minute()<10)Serial.print(0); Serial.print(datetime.minute(),DEC); Serial.print(":"); if(datetime.second()<10)Serial.print(0); Serial.println(datetime.second(),DEC); } // Temperatur ausgeben void show_temperature(float temp){ Serial.print("Temperatur: "); Serial.print(temp); Serial.print(" "); // Hier müssen wir ein wenig tricksen Serial.write(176); // um das °-Zeichen korrekt darzustellen Serial.println("C"); }
start the serial monitor and then we should get the following output:
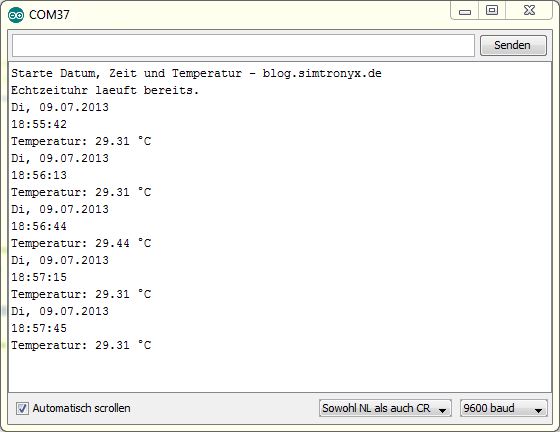
DS1307 RTC and DS18B20 temperature measurement – Serial Monitor
Postgraduate project with the DS1307 RTC module:
Simple indoor climate monitoring with an Arduino, BMP085, DHT11 and a RTC
Components:
eBay: | Breadboard jumper wires RTC Module |
Amazon: | RTC Module |
Good?











